Towards mapping unknown environments with a robot swarm
Mapping is an essential task in many robotics applications. A map is a representation of the environment generated from robots positions and sensors data. A map can be either used to navigate the robot that built it, or shared with other agents: humans, software, or robots. To build a map, it is frequently assumed that the positions of the robots are a priori unknown and need to be estimated during operation. Accordingly, the problem that robots must solve is known as simultaneous localization and mapping (SLAM). This problem has been extensively studied in the past decades. As a result, a large number of methods have been developed to generate various types of maps, in different environments, and using data gathered by a broad range of sensors. However, most of these methods were conceived for single-robot systems. Multi-robot SLAM is a more recent research direction that addresses the collective exploration and mapping of unknown environments by multi-robot systems. Yet, most results so far have been achieved for small groups of robots. Multi-robot SLAM is still a growing field, and a number of research directions are yet to be explored. Among them, swarm SLAM is an alternative, promising approach that takes advantage of the characteristics of robot swarms.
A robot swarm is a decentralized multi-robot system that can collectively accomplish missions that a single robot could not accomplish alone. With respect to centralized multi-robot systems, robot swarms present unique characteristics. First, a swarm does not need global knowledge nor external infrastructure to operate, and robots in a swarm only interact with close peers and the neighboring environment. This allows robot swarms to comprise an arbitrarily high number of robots without decreasing their performance (scalability). Then, as swarms are decentralized and self-organized, individual robots can dynamically allocate themselves to different tasks and hence meet the requirements of specific environments and operating conditions, even if these conditions evolve at operation time (flexibility). Finally, a robot swarm is characterized by high redundancy resulting from the large number of robots composing it. Redundancy, together with the absence of centralized control, allows robot swarms to cope with the loss or failure of some robots, and also with noise thanks to redundancy of measurements (fault tolerance). Hence, locality of sensing and communication, self-organization, and redundancy enable desirable properties such as scalability, flexibility, and fault tolerance that make a robot swarm the ideal candidate to perform missions in large unknown environments in which the risk that individual robots fail or are lost is high.
It is our contention that robot swarms could perform SLAM in environments and under operating conditions that are not appropriate for individual robots and for centralized multi-robot systems. Indeed, the robots in a swarm can work in parallel and thus quickly cover large areas. This is especially useful in dynamic environments in which changes can occur unexpectedly. By exploring in parallel, robots could track changes in the environment, identify areas that evolve more rapidly, and autonomously allocate more resources (i.e., more robots) to these areas. Thanks to its fault tolerance, a robot swarm can also operate in dangerous environments—like sea depths or outer space—as loosing a few robots will have little impact on the mission. This is also true cost-wise as robots in a swarm are often relatively simple and cheap in comparison with other robotics systems. However, a limitation is that simple robots usually rely on low-quality sensors and therefore, at the moment, swarm systems cannot produce metric maps as precise as those produced by single robots and centralized multi-robot systems. Yet, one of the main interests of robot swarms lies in their capacity of covering quickly large areas. Hence, they are best suited for building abstract maps in time-constrained scenarios. Indeed, applications requiring a very precise map are typically not constrained by time, while time-constrained applications can cope with rough but informative maps. For example, a patrolling robot has sufficient time to build a complete map of the building it is supposed to protect before beginning its protection task. On the other hand, robots sent to explore a disaster area and to locate survivors can quickly give to the rescuers an approximate path to the victims location.
At the moment, swarm robotics research has achieved many significant results but lacks proper applications. As building maps is at the basis of many robotics behaviors, swarm SLAM is a step forward to deploy robot swarms in real world scenarios. We believe that it could play an important role in time- or cost-constrained scenarios or for monitoring dynamic environments.
Our research in swarm SLAM is supported by the European Research Council (grant agreement No 681872) and the Belgian Fonds de la Recherche Scientifique–FNRS.
‘Neutrobots’ smuggle drugs to the brain without alerting the immune system
A robot that senses hidden objects
ThermoBots: Microrobots on the water
JAX vs Tensorflow vs Pytorch: Building a Variational Autoencoder (VAE)
Even without a brain, these metal-eating robots can search for food
Scientists create the next generation of living robots
Case Study: Grippers from Zimmer Group Automate a Cleaning Machine for Sterile Glass Vials
Roboreptile climbs like a real lizard
Ready for duty: Healthcare robots get good prognosis for next pandemic
Redefining Communications for Today’s Mobile Workforces
Boston Dynamics’ new robot Stretch can help move boxes in warehouses
#331: Multi-Robot Learning, with Amanda Prorok
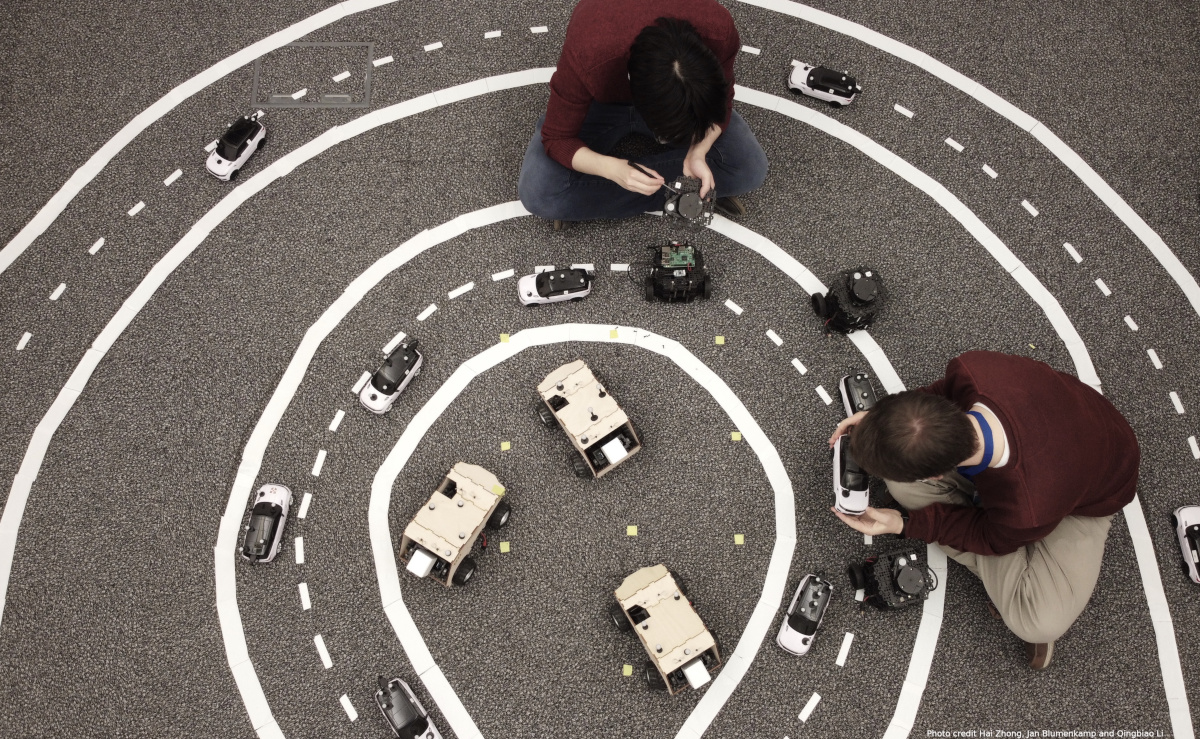
In this episode, Lilly interviews Amanda Prorok, Professor of Computer Science and Technology at the University of Cambridge. Prorok discusses her research on multi-robot and multi-agent systems and learning coordination policies via Graph Neural Networks. They dig into her recent work on self-interested robots and finding explainability in emergent behavior.
Amanda Prorok
Amanda Prorok is an Assistant Professor (University Lecturer) in the Department of Computer Science and Technology, at Cambridge University, and a Fellow of Pembroke College. She serves as Associate Editor for IEEE Robotics and Automation Letters (R-AL) and Associate Editor for Autonomous Robots (AURO). Prior to joining Cambridge, Prorok was a postdoctoral researcher at the General Robotics, Automation, Sensing and Perception (GRASP) Laboratory at the University of Pennsylvania, USA, where she worked with Prof. Vijay Kumar. She completed her PhD at EPFL, Switzerland, with Prof. Alcherio Martinoli.
Links
- Download mp3 (19.6 MB)
- Subscribe to Robohub using iTunes, RSS, or Spotify
- Support us on Patreon